❯ Mobile Security Notes
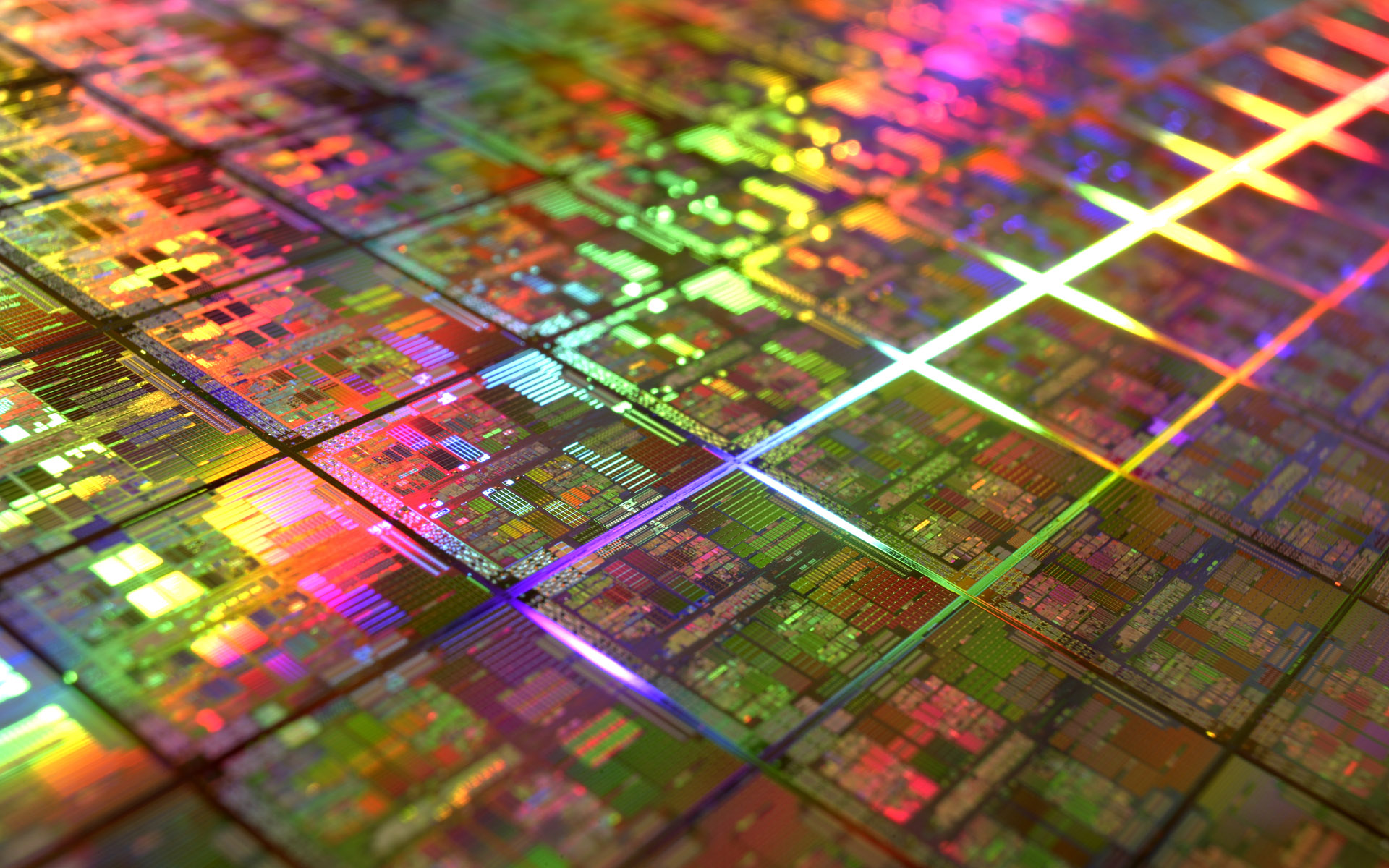
Table of Contents
Android Framework
-
Linux Kernel (Layer 0)
- Allows for permissions model
- Multi user OS
- Process isolation
- Extensibility mechanism for secure IPC
- Ability to remove unnecessary and insecure parts of kernel
-
Hardware Abstraction Layer (HAL) (Layer 1)
- Facilitates the interaction between software and hardware components
- Used to interact with the physical sensors and devices: Bluetooth, Wi-Fi, Camera
-
Native Libraries (Layer 2)
- Contain important libraries which are used by the services and application
- Eg:
- SSL
- SQLite
- WebKit (browser)
- OpenGL (graphics)
-
Android Runtime (Layer 2)
- Dalvik VM (Replaced by Android Run-Time)
- Bulk of android executed by Dalvik.
- Designed with mobile devices in mind and cannot run Java bytecode
.class
directly- Dalvik reduces memory footprint via increased sharing
- Dalvik inputs
.dex
files which maybe packaged inside Java libraries or APKs - Each application runs in its own VM
-
Application Framework (Layer 2)
- Native APIs for Android system for accessing resources
- Helping the developer develop functionalities pertaining to the resources available in a phone
-
Applications (Layer 3)
- End user application which uses the layer below it.
- All the system and user applications reside in this layer
Android Application Architecture
[Java Source Code] ----------------> [Java Bytecode] ------> ((JVM))
<Java Compiler>
- Converted to Java Bytecode so that any environment can run it
-
Dalvik VM required for running Android applications. It is built-in in mobile phones
-
Dalvik VM replaced by Android Run-Time
-
AAPT: Android Asset Packaging Tool. Packages
-
Dalvik VM
- Plain Java code is compiled Just-in-time to bytecode during runtime on the machine.
- Leads to slowdown as compilation during runtime is time-consuming
.dex
: Android’s compiled code file. These.dex
files are zipped into a single.apk
file.odex
: Created by the OS to save space and increase the boot speed of an app- dexopt is used to optimize DEX to ODEX which contains the optimised bytecode
-
Android Run Time
- During installation, the whole code is converted into native code and executed natively
- Downside is that more storage is required to store the compiled binaries
- Uses the same
.dex
files - ART compiles apps using the
dex2oat
tool. DEX files are accepted and compiled app is generated
DVM | ART |
---|---|
Faster boot time | Rebooting is longer |
Cache builds up overtime | Cache built up in the first use |
Occupies less storage space | More internal storage is used |
Longer app loading | Faster and smoother app loading and processing |
Understanding APK Internals
AndroidManifest.xml
: Contains the package name, minimum API version, App config, User-Granted Permissionsassets/
: Directory containing the app assetsclasses.dex
: Classes compiled in DEX file formartlib/
: Third party libraries not included in Android SDK; Dependenciesres/
: Contains resources that haven’t been compiled intoresources.arsc
resources.arsc
: Precompiled resources, such as XML filesMETA-INF/
: Contains the app’s metadata:MANIFEST.MF
CERT.SF
CERT.RSA
Android Application Components
- Application is a collection of components.
- All these are Java Classes
- These application components are just running inside Java Runtime.
- Activity: A screen that has a user interface. Handles the user interaction and contains the UI/UX. Eg: An application’s UI with the home screen, nav bar, profile page etc.
- Ensures only one activity gets user input at a time. Other applications can’t grab the screen of your bank application.
- Service: An application component that performs long running tasks in the background. Doesn’t have user interface.
- Eg: Music player service playing music in the background;
- Browser downloading a file in the background
- Broadcast Receiver: A component that listens to system-wide announcements. Just like services but for long running tasks.
- Eg: Battery check/Notification; Phone call.
- Content Provider: Application component for storing and sharing data efficiently. Sandboxing means application data is isolated from other applications. Content Provider acts as a middle-man for sharing and accessing this data
- App data being stored in a SQLite Database; One app accessing the files and photo gallery.
- Intent: Asynchronous messaging object for communication between application components. Used for communication between application components of same or different applications. Intents handle this communication between application components.
- A structured message containing
- Component: to which to send a message.
com.android.dialer/Dial
=java.app.name/name_of_component
- Action: the action which you want the component to take
android.intent.DIAL
: A predefined string/intent to perform the action- To view an object,
android.intent.VIEW
.. etc
- Data/MIME: To send to the component
- URI of the resource to send to data
tel://9956668942
http://resource.com
- Component: to which to send a message.
- Explicit Intents: Component is specified. ie, the sender knows which application or service receives the intent. Hence, it’s upto the receiver to accept or reject the intent and to appropriate permission checks
- Implicit Intents: Component is not specified and the Reference Manager is the one responsible to find an application suitable for handling the message.
- A structured message containing
- Manifest:
- XML File
- Describes the components
- Describes how other parts of the system should interact with an application
- Consists of Labels too.
Labels and Reference Monitor
-
Labels define and describes the privileges and permissions an application. Java style domain names used to define the permission strings.
-
Labels are described in
manifest.xml
and on runtime, are dependent on the user to grant the requested label permissions. -
An application may have a privilege with the label “VIEW_FRIENDS” that allows you to view the location of your friends.
-
To enforce the privilege, the Content Provider (database storing the location of friends) would have the “VIEW_FRIENDS” label attached to it. Anyone that wants to access the database has to have the label
-
A label = UID/GID but arbitrary strings
-
“VIEW_FRIENDS” = com.google.app.VIEW_FRIENDS (Java Style domain of the application defining it)
-
Types of Labels
- Normal (permission): User doesn’t get prompted to grant this label and the application just gets the label. Permission granted/rejected automatically so that the user doesn’t make mistakes in granting or rejecting the permission
- Dangerous:
- Signature: Accessible to the applications signed with the same developer key as the application requesting it. Done for the operations between the same applications from same developer. Tag exists so that the user isn’t prompted and has less chance of making mistakes or being tricked into granting/rejecting permission
-
Reference Monitor: Mediates all intents requests.
- If App1 wants to send message to App2, Reference Monitor figures out where to relay the message.
- In charge of checking of Labels and Permissions.
- Stores a list of installed applications and the labels corresponding to all the applications
- Label Checks in App1: Cannot trust the sender. Could be malicious. Can’t guarantee to do the correct checks
- Label Checks in App2: Want to shift the burden of having bug-free, secure label checking from the developer to the system.
- Easier to do the checks in one place. Hence, Reference Monitor is the one responsible for the checks.
- Handles Implicit Intents and takes permission into account to find suitable application for that message. Eg. A private PDF_VIEW message for viewing PDFs
- TImes when reference monitor isn’t needed
- Network access
- Filesystem + SD Card access
- Hardware access
- Android translates the label strings by setting UIDs/GIDs and policy of the resources.
[APP1] [APP2] [Reference Monitor]
| |
| |
->------------------------>--
intent from APP1 to APP2 thru RM
-------------------------------
| Running on Linux Kernel |
Application Sandbox & Permissions
Android Application Sandbox
- Takes advantage of Linux user-based protection to identify and isolate app resources. Linux UIDs and GIDs are asssigned to each application based on the permission and roles
- Each application runs in its own process
- Security enforced thru UID/GID and file permissions
- UID stored in
/data/system/packages.list
- Each application alloted a private directory
/data/data/<package.name>
- By default, apps cannot interact with each other and have limited access to the OS
- To provide access and functionalities, Androis allows applications to request fine grained access rights known as permissions
- The permissions an application can request is stored in AndroidManifest.xml file
<uses-permission android:name="android.permission.SEND_SMS"/>
Permissions
- These are an effective method for access control between applications.
- Install time: Give your app limited access to restricted data. These permissions are automatically granted to the app
- Normal permissions: Allow access to data and actions that extend beyond the app’s sandbox
- These data and actions present very little risk to the users
android.permission.VIBRATE
android.permission.FLASHLIGHT
- Signature permissions: For a signature permission, two apps signed by the same certificate are granted access to each other’s components.
- Automatically granted to the app
- Normal permissions: Allow access to data and actions that extend beyond the app’s sandbox
- Runtime / Dangerous permissions: Give the app additional access to restricted data and ability to perform restricted actions
- These permissions have to be explicitly granted to the app
android.permission.MODIFY_PHONE_STATE
android.permission.SET_PROCESS_LIMIT
- Insecure permissions:
mode_world_readable
&mode_world_writable
- These are deprecated permissions and cannot be used in API Levels 17+. These permissions should be strictly avoided
mode_world_readable
: Allows all other applications to have read access to the created file- Using this permission is very dangerous and will likely cause security holes in applications
- Allows any application the ability to read any and all potentially sensitive information in the file
- In the event of a security compromise, this can also lead to Sensitive Data Exposure
mode_world_writable
: Allows all other applications to have write access to the created file- Allows any application the ability to write to or overwrite critical data and files
- In the event of a security compromise, the attack can inject code and escalate privileges
Rooting
- Rooting: The process of allowing users of Android to attain privileged control of various Android subsystems. Rooting gives access to superuser permissions like on any other Linux based OS
- The process involves installing the
su
binary on the system or replacing the system entirely with a rooted custom ROM. - Bootloader access is the most difficult and important part of rooting an android device.
- Once that is unlocked, a recovery image is flashed
- After this, the custom ROM can be loaded and flashed onto the system partition
- The process involves installing the
- Obfuscation
- Cryptography etc
- Proguard Dexguard
Cryptography and Implementations
- Symmetric Key Cryptography
- Only one key used to encrypt and decrypt information on both sides
- Security dependant on the one cipher key which both the sender and reciever should have
- Security problem posed by securely sharing the key
- Resource utilisation or computation required is much lower
- Hence, it’s used to transfer large amount of data at fast speeds
- Encryption and keys are based on substitution and permutation and XOR etc, hence keys are easier to derive
- Asymmetric Key Cryptography
- A pair of keys; public/private keys are used to encrypt and decrypt
- Public key is available to everyone, private key is kept stored safely
- Once cannot derive the private key from public key
- No problem faced in secure key sharing
- Resource utilisation is much higher
- Requires more space to transfer
- Requires more time to compute since core logic is based on elliptic curves and modulo arithmetic
Password Hashing/Storage and Digital Signature
- Hash: A function that can be applied to any block of data and produces a fixed-length, randomly generated output which is infeasible to reverse.
- It is theoretically infeasible to generate a message back from its hash value mathematically
- Brute forcing the only option and that is impractical
- The same message always results in the same hash and a small change in the message results in a large change in the hash
- Collision free:
h(x) != h(x')
Storing a Password
-
Generate long random salt using Cryptographically Secure Pseudo-Random Number Generator (CSPRNG) for each entry
- CSPRNG is important to generate a secure random salt
- This is because a static salt being used acts as a single point of failure. Any password / hash being leaked could also lead to the salt being leaked. Bruteforcing the passwords and hashes are a possibility through rainbow tables
-
Prepend it to the password and hash it with argon2, bcrypt, scrypt, PBKDF2
-
Save both the salt and hash in the database
-
Rainbow Tables: Precomputed table for caching the output of hash functions, usually for cracking salted hashed passwords.
- This is prevented by the use of cryptographic salts
- A salt is a random data that is used as an additional input into a hash function
saltedhash(password) = hash(password + salt)
saltedhash(password) = hash(password) + salt
- Salt value has to be generated randomly during password storage
- This is prevented by the use of cryptographic salts
-
PBKDF2: Refers to Password Based Key Derivation Function 2 and is resistant to rainbow table and brute force attacks
- This function applies a pseudorandom function (HMAC) to the input password along with salt to produce a derived key
key = pbkdf2(password, salt, iterations-count, hash-function, derived-key-len)
password
– array of bytes / string, e.g. “p@$Sw0rD~3” (8-10 chars minimal length is recommended)salt
– securely-generated random bytes, from CSRNGiterations-count
, e.g. 310000 as per OWASP 2021hash-function
for calculating HMAC, e.g.SHA256
derived-key-len
for the output, e.g. 32 bytes (256 bits)
- If the result isn’t used as a cryptographic key but rather a hash value, then it’s called a password hash
- Password hashes are different (more safe from brute forcing or rainbow tables) as they contain a work factor ie the iterations
Validating Password
- Retrieve the salt and the hash
- Prepend the salt to the entered password and hash
- Compare it with the hashed stored in the database
Difference between Random and Secure Random
java.util.Random |
java.security.SecureRandom |
---|---|
Not cryptographically strong, based on a random number algorithm which can easily be broken | Generates a CSRN complying with FIPS standard |
Size is 48 bits | Size can be upto 128 bits |
The unix system clock is used for seed generation | The entropy of the system is used as seed (/dev/random ) etc |
Bruteforcing is much easier 2^48 | Bruteforcing much more impractical since 2^128 |
Not crypto secure | Crypto secure |
Key Derivation and Management
-
It is recommended that the application should not store sensitive data unless it absolutely has to
-
Confidentiality: AES-GCM-256 or ChaCha20-Poly1305
-
Integrity: SHA-256, SHA-384, SHA-512, Blake2
-
Digital Signature: RSA (3072 bits and higher), ECDSA with NIST P-384
-
Key Establishment: RSA (3072 bits and higher), DH (3072 bits or higher), ECDH with NIST P-384
-
Android Keystore: The Android Keystore system lets you store cryptographic keys in a container to make it more difficult to extract from the device. Once keys are in the keystore, they can be used for cryptographic operations with the key material remaining non-exportable. Moreover, it offers facilities to restrict when and how keys can be used, such as requiring user authentication for key use or restricting keys to be used only in certain cryptographic modes
- PKCS#12 defines an archive file format for storing many cryptography objects in a single file
iOS
iOS Architecture
- Layered architecture. iOS works as an intermediary between the underlying hardware and the apps you make
- The layers between the application and hardware layer do the job of communication
- Framework: A directory that holds dynamically shared libraries like
.a
files, header files, images and helper apps that support the library.- Every layer has a set of framework which the developer uses to construct the applications
- Core OS: Holds the low level features that the other technologies rely on or are built upon:
- Core bluetooth framework
- Accelerate framework
- External accessory framework
- Security services framework
- Local authentication framework
- Core Services: Some crucial and important frameworks are present in this layer.
- Address book framework; Cloud Kit framework; Core data framework; Social framework
- Media Layer: Graphics, video and audio is enabled
- Core graphics framework; Core Animation framework
- Cocoa Touch: This is the application layer acting as an interface for the user to work with the OS.
- EventKit framework; GameKit; MapKit iOS Application Structure
iOS Apps Execution Stages
- Not Running: App has not been launched yet or was terminated by the system
- Inactive: The app is running in the foreground but is not recieving any events
- Occurs when the app is transitioning states
- Not Running -> Inactive -> Active
- Active -> Inactive -> Background
- Background -> Inactive -> Active
- Occurs when the app is transitioning states
- Active: Main execution state of an application
- Background: App is in background, is still executing code and receiving events (not UI events)
- Suspended: App is in backgroun but not executing code. App remains in the memory
iOS Sandboxing
- An iOS Sandbox has three containers;
- Bundle Container:
MyApp.app
- Data Container: Documents; Library; Temp
- iCloud Container
- Bundle Container:
- IPA: iOS App Store Package tree
- Payload
MyApp.app
: Bundle Container- Application Binary: Encrypted;
- Mobile Provision File: Details of the UD/IDs of
- Code Signature
- Bundled Resource Files: Resources reqd to build the application
- iTunesArtwork
- iTunesMetadata.plist
- Payload
Jailbreaking iOS
- Gaining root access on Apple device. Best approach for security testing an application
- Allows for
- Removing security limitations on the OS
- Installing important software tools
- Cydia impactor
- Checkrain
- Pangu
- Uncover
- Access to Objective-C Runtime
- Types of Jailbreaks
- Untethered: No consequences on reboot
- Semi-tethered: Less consequences on reboot
- Tethered: Complete loss of jailbreak on reboot
OWASP Top 10 Mobile
- Improper Platform Usage
- Misuse of platform feature or failure to use platform security controls
- Android intents
- Platform permissions
- Misuse of Touch ID
- Misusing the platform security controls
- Insecure Data storage
- Insecure storage of sensitive data like;
- Usernames
- Credentials
- Pasteboard data (side channel data)
- Location data
- Cached application messages or transaction history
- PII
- Places it can be insecurely stored
- SQLite DBs
- Plist Files
- Log Files
- XML Stores
- SD Card
- Keychain
- Custom Files
- Cookie stores
- Insecure storage of sensitive data like;
- Insecure Communication
- Communication over insecure channel. Poor handshaking, incorrect SSL versions, Weak negotiation
- Leads the risk of:
- Exposing user data
- Session IDs
- Tokens
- Business logic flaws
- Account theft
- Data coming to the device
- Data going to server
- Insecure Authentication
- Risk
- Absence of proper authentication mechanisms
- Failure to identify user or maintain the identity
- Account takeover
- Bypassing
- Extracting sensitive user information
- Occurs when
- App is executing backend API without access token
- App is storing password or pin in local data storage
- Weak password policy
- Risk
- Insufficient Cryptography
- Refers to sensitive data assets where cryptography was attempted and wasn’t done correctly
- Risk/Impact
- Privacy violation
- Information theft
- Code theft
- Reputational damage
- Mitigation
- Make sure correct implementation
- Proper key storage and management
- Not implementing weak algorithms
- Insecure Authorization
- Occurs when access control roles are not configured properly
- Risk
- IDOR
- Hidden endpoints (backend endpoints)
- User roles or permissions
- Client Code Quality
- Code level implementation problems in the mobile client
- Risk
- Buffer overflow
- Using the wrong API
- Code mistakes
- DOM based XSS in WebView
- Mitigation
- Maintain consistent coding patterns
- Follow secure coding practices
- Source code reviews
- Code Tampering
- Covers smali patching, local resource modification and method hooking
- Technically all mobile is vulnerable
- Risk
- Unauthorized new features
- Loss in piracy, business logic bypass
- Mitigation
- Root detection
- Reverse Engineering
- Includes analysis of final core binary to determine its source code, libraries, algorithms
- Reveals information about backend servers and other sensitive data
- Extraneous Functionality
- Developer hidden backdoor functionality which is not intended to be released on a production environment
- Eg; hardcoded passwords and credentials
- Enable logging (sensitive)
- Disable MFA
Android and iOS WebViews
setAllowContentAccess
- Default is True
- Allows WebView to access content providers
setAllowFileAccess
- Default is True
- Allows WebView to load content from filesystem
file://
schema
setAllowFileAccessFromFileURLs
- Default is False since API 16
- Allows JavaScript in WebView to access any resources from filesystem using
file://
setAllowUniversalAccessFromFileURLs
- Default is False since API 16
- Allows JS from local web pages that are rendered inside WebViews and called with a
file://
schema to access resource from any origin.